Adding simon.js to your site
Adding the following code (known as the "JavaScript tracking snippet") to your site's templates is the easiest way to get started using Simon.js.
<script>
var _sd = _sd || {};
_sd.partnerId = 'YOUR_PARTNER_ID_HERE';
_sd.domain = 'YOUR_DOMAIN_HERE';
_sd.environment = 'YOUR_ENVIRONMENT_HERE';
_sd.debug = true;
_sd.behaviors = {};
(function(s,i,m){
w=window;d=document;n='script';l=Array.prototype.slice;
w._sd.lib=i;w.SimonData=s;
w[s]||(w[s]={
send:function(){(w[s].q=w[s].q||[]).push(arguments)},
identify:function(){a=l.call(arguments);a.unshift('identify');w[s].send.apply(null,a)},
track:function(){a=l.call(arguments);a.unshift('track');w[s].send.apply(null,a)}
});
t=d.createElement(n);t.src=i;
o=d.getElementsByTagName(m)[0];o.parentNode.insertBefore(t,o)
})('sd','LOCATION_OF_SIMON_JS','script');
</script>
The code should be added before the closing tag, and the strings YOUR_PARTNER_ID_HERE
, YOUR_DOMAIN_HERE
, YOUR_ENVIRONMENT_HERE
and LOCATION_OF_SIMON_JS
should be replaced with their corresponding settings for your organization. To see these values, from Simon expand Admin Center then click Signal Installation:
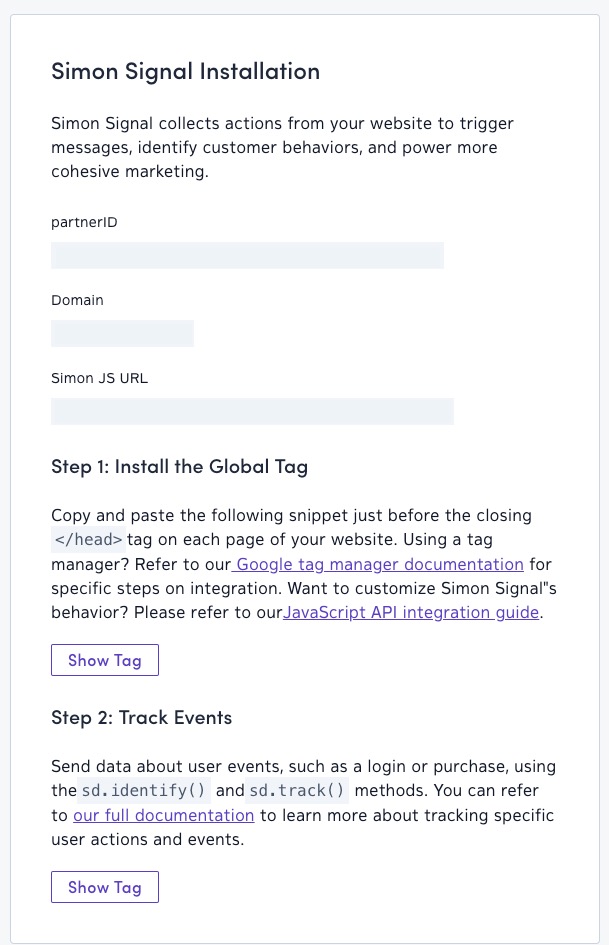
The options available to configure simon.js with are the following:
Parameter | Description |
---|---|
partnerId | The internal partnerId Simon uses to identify the customer's site. |
domain | The domain which the cookies for simon.js should be set under. (defaults to the domain of the current page). For example to put simon.js on all of simondata.com (including subdomains) the value should be .simondata.com |
environment | The Simon environment the site is going to send data to. (defaults prod). It can be one of the following: local, staging, or prod |
behaviors | Optional configuration that augments the default behavior of simon.js Supported: v2.0+ |
behaviors.trackPageViewOnLoad | Default to false . When set to true , page_view events will be captured automatically when a page loads.Supported: v2.0+ |
debug | A setting for development which will send information to the console about any errors and the status of simon.js (defaults to false) |
LOCATION_OF_SIMON_JS | The URL where simon.js is hosted. |
If you do not know your partner ID, please contact us.
Sending clickstream data to Simon Data
Exceptions and debug mode
If you're unsure about whether your event is correctly formatted, you can use the debug mode. Exceptions will be silent unless the debug mode is active. Check out the details below.
The identify command
sd.identify(userId, [traitsObject])
A note on
userId
The
userId
is intended to be your custom user identifier, e.g. a primary key on your production users table. However, despite being a positional parameter in the identify call, it is not required. If you don't have auserId
or don't want to pass one, set the first positional parameter to an empty string.Do not replace
userId
with e.g. an email address as this can cause undesirable consequences in identity management downstream.
This is an example of an identify
call:
sd.identify('97980cfea0067', {
email: '[email protected]',
name: 'Joe Smith',
firstName: 'Joe',
lastName: 'Smith',
userId: '97980cfea0067',
username: 'joesmith',
properties: {
campaignId: '12345'
}
});
A call to identify
establishes the identity associated with a userId
. The variable userId
is a user identifier that you specify. identify
can be called to pass information when a source of identity is recognized, such as an authenticated login, a click from an email with an identity URL parameter, and signup for newsletter subscription. Different types of identifiers can be passed on.
When a user registers with you for the first time, you should call identify
and also make a separate call to registration
.
Once identify
has been called during a session, the payload for subsequent calls to track
(see below) will include the supplied traits for the duration of that one browser session.
Value | Type | Required | Description |
---|---|---|---|
String | Yes* (see below) | The email address of the user (e.g. [email protected]). | |
firstName | String | No | The first name of the user (e.g. Joe). |
lastName | String | No | The last name of the user (e.g. Smith). |
name | String | No | The full name of the user. (e.g. Joe Smith). |
userId | String | No | The user id. (e.g. 97980cfea0067). |
username | String | No | The username (e.g. joesmith). |
properties | Object | No | An object of extra arguments (e.g. { campaignId: '12345' }). |
Next-Gen Identity Identifier Requirements
If your organization is on Next-Gen Identity, email address is no longer required. However, you must ensure that the identifier values present on your Simon Signal events match the identifiers present in your organization's Simon account.
For example: if your account utilizes the User ID identifier and you want to include userId on your Simon Signal events, you must pass us the actual User ID values in the userId field in Signal events and not the values of another identifier.
If there is a non-user Id field you want to include in your Signal events, we recommend putting that value in the 'properties' object.
Adding channel identifiers to identify
identify
You can use the properties
object to specify specific channel identifiers to be used by Simon. These follow the following syntax: properties.<channel key>.<identifier key>
, as highlighted in the example below.
Channel Name | Channel key | Identifier key |
---|---|---|
Salesforce Marketing Cloud | sfmc | primarySubscriberKey |
sd.identify('97980cfea0067', {
email: '[email protected]',
userId: '97980cfea0067',
properties: {
sfmc: {
primarySubscriberKey: '[email protected]'
}
}
});
The track command
sd.track(eventType, [eventObject]);
This is an example of a Product View event:
sd.track('product_view', {
productId: '632910392',
brand: 'Acme',
category: 'Apparel/Men/T-Shirts',
productName: 'Nyan Cat T-Shirt',
price: 24.95
});
A track
command may be invoked to pass user-interaction data to Simon Data. These user-interactions are captured by specific events that are documented in this API and are explained in more detail below. Each event has an Event Type specifying the name of the event and an associated Event Object which contains additional metadata about the event. If identify
(see above) has been called earlier during a session, track will include the traits supplied to identify
. In all cases track
will include the clientId
, i.e. the cookie for that browser.
Parameters
The properties that can be specified within the eventObject
object literal vary depending on the event type.
Using custom properties
The event schema is enforced and events that are missing required properties or include unrecognized properties will be rejected during processing. Using the debug mode during your integration set-up will let you know that this is happening. However, all event types support custom properties within the
properties
part of the payload (see an example for product view events). The API also supports custom events if there is not a pre-existing matching event type, but make sure to adhere to the schema for those events, too.
Event Type | Event Object |
---|---|
page_view | none |
product_view | productId variant productImageUrl productUrl brand category color productName size style price properties |
add_to_cart | productId variant quantity productImageUrl productUrl brand category color productName size style price properties cartItems |
cart | cartItems productId variant quantity productImageUrl productUrl brand category color productName size style price properties |
update_cart | productId variant quantity productImageUrl productUrl brand category color productName size style previousQuantity price properties cartItems |
remove_from_cart | productId variant quantity productImageUrl productUrl brand category color productName size style price properties cartItems |
complete_transaction | transactionId revenue cartItems productId variant quantity productImageUrl productUrl brand category color productName size style price properties shipping tax promotion properties |
registration | email username userId optIn firstName lastName properties |
favorite | productId variant productImageUrl productUrl brand category color productName size style price properties |
waitlist | productId variant quantity productImageUrl productUrl brand category color productName size style price properties |
authentication | userId isLoggedIn ARN properties |
custom | eventName properties |
Debugging
Enabling debug mode
Debug mode can be enabled by setting the debug
parameter to true
when adding the tag.
Add the setting to the tag to enable debug mode:
_sd.debug = true;
Exceptions are silent by default
By default, exceptions are caught silently and send to our servers for further analysis. Enabling debug mode will allow exceptions to print to the console without being sent to our servers.
Understanding server responses
Add to cart event missing productId:
sd.track('add_to_cart', {
variant: '808950810',
quantity: 1,
brand: 'Acme',
category: 'Apparel/Men/T-Shirts',
color: 'Black',
productName: 'Nyan Cat T-Shirt',
price: 24.95,
properties: {
desc: 'Slim cut t-shirt with a cat on it'
}
});
If the implementation of simon.js is healthy, all XHR requests should complete with a 200 (OK) or 204 (No Content) response code. Our servers will respond with a 412 (Precondition Failed) response code in the case that a given request does not meet our criteria and debug mode is on.
Error Response Code | Action |
---|---|
412 | You are sending invalid data. Check the response body for more specifics. |
403 | Your domain is not recognized, but the data is in a valid format. If you are connecting from a development or staging environment, set _sd.environment = 'staging' while testing. If you are seeing this issue from your production environment, please contact us. |
500 | Contact Simon Data |